Oh noes!
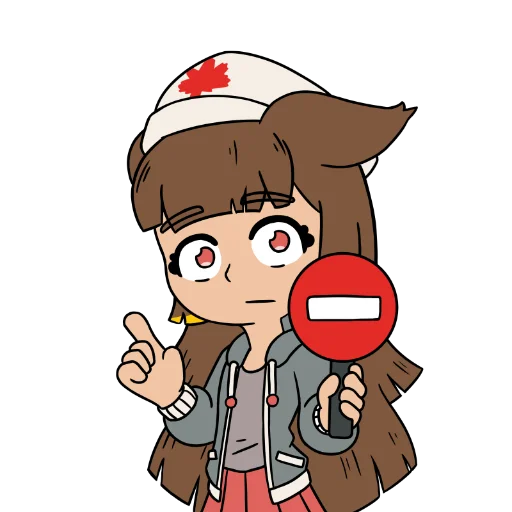
Access Denied: error code 8e399aa8f9f5d95a8cd0ad01284ea904784a854e9c6ab17ac9994f479d18aaed.
Go home or if you believe you should not be blocked, please contact the webmaster at lumi@shad.moe
Access Denied: error code 8e399aa8f9f5d95a8cd0ad01284ea904784a854e9c6ab17ac9994f479d18aaed.
Go home or if you believe you should not be blocked, please contact the webmaster at lumi@shad.moe